How to Generate CSV Files from JSON Data in PHP
Data transformation between different formats is an essential process in software development, allowing for compatibility across systems and applications.
One such scenario involves transforming JSON data into CSV format. CSV (Comma-Separated Values) and JSON (JavaScript Object Notation) are two widely used formats for representing structured data, each with distinct advantages.
CSV excels in simplicity and compatibility with spreadsheets and databases, while JSON has more complex data structures and flexibility.
In this guide, you will learn how to generate a CSV file from JSON data using PHP, in a step-by-step process, with the help of examples.
Steps to Convert JSON to CSV with PHP
Follow the steps below to generate a CSV file from JSON data in PHP.
Step 1: Reading JSON Data
To start, you need to read the JSON data into your PHP script. Use the json_decode()
function to convert the JSON string into a PHP associative array.
$jsonData = '{"name":"John Doe", "age":30, "city":"New York"}';
$dataArray = json_decode($jsonData, true);
In case your data is stored in a JSON file instead of a variable, use the file_get_contents()
function to read it.
$jsonData = file_get_contents("path/to/file.json");
$dataArray = json_decode($jsonData, true);
Step 2: Creating the CSV File
The fopen()
function is used to open a file. The first argument specifies the file name (and path if in a different directory from the PHP script) and the second, the access mode in which to open the file. To write to the file, use the write (w
) mode.
If the file does not exist, a new file with that name gets automatically created and opened in write mode.
$csvFile = fopen('users.csv', 'w');
Step 3: Adding Data to the CSV File
CSV data is displayed with a header row, which is the first row/line in the document, comprising column headings, and the other rows comprising the actual data.
From the JSON data given in step 1 above, we would have a CSV file with a header row comprising three columns ("name", "age", and "city"), and one row of data in respective columns as ("John Doe", "30", and "New York").
Therefore, when adding data to our CSV file, we need to use that format. We start by adding the header row (array keys) and then followed by the actual data (element values). Both the two types of records are added using the fputcsv()
function.
The first argument specifies the open file that we want to write to while the second specifies an array of data to write to the file.
// Writing CSV header
fputcsv($csvFile, array_keys($dataArray));
// Writing data to the file
fputcsv($csvFile, $dataArray);
Step 4: Closing the file
Lastly, close the file using the fclose()
function. Generally, it's always a good idea to close a file when you're done with it to properly release the resources associated with it and ensure its integrity by preventing anything that could corrupt it.
// Closing the CSV file
fclose($csvFile);
Example 1
<?php
$jsonData = '{"name":"John Doe", "age":30, "city":"New York"}';
$dataArray = json_decode($jsonData, true);
$csvFile = fopen('users.csv', 'w');
fputcsv($csvFile, array_keys($dataArray)); // Write CSV header
fputcsv($csvFile, $dataArray); // Write data
fclose($csvFile);
?>
When the above script is executed, it generates a file named 'users.csv' in the same directory with the content below:
name,age,city
"John Doe",30,"New York"
When opened with Microsoft Excel looks like the below screenshot:
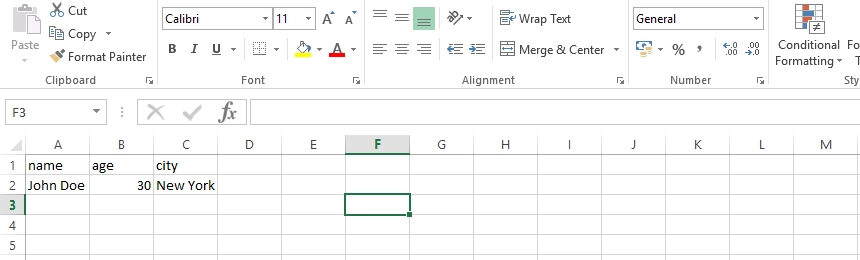
Example 2
Generating a CSV file from a JSON string comprising an array of objects.
<?php
// Sample JSON data
$countriesJson = '{
"countries": [
{
"name": "United States of America",
"code": "US",
"city": "New York"
},
{
"name": "India",
"code": "IN",
"city": "New Delhi"
},
{
"name": "China",
"code": "CN",
"city": "Beijing"
},
{
"name": "Germany",
"code": "DE",
"city": "Berlin"
},
{
"name": "Kenya",
"code": "KE",
"city": "Nairobi"
}
]
}';
// Decoding JSON data to an Array
$countriesArray = json_decode($countriesJson, true);
// Creating and opening a new CSV file
$csvFile = fopen('countries.csv', 'w');
/**
* Writing CSV header row comprising of array keys from
* the first object of the 'countries' object
**/
fputcsv($csvFile, array_keys($countriesArray['countries'][0]));
/**
* Writing the data rows in the CSV file by looping through
* the array of objects in the 'countries' object
**/
foreach ($countriesArray['countries'] as $row) {
fputcsv($csvFile, $row);
}
// Closing the CSV file
fclose($csvFile);
?>
The above script when executed generates a CSV file named 'countries.csv' in the same directory, with these contents.
name,code,city
"United States of America",US,"New York"
India,IN,"New Delhi"
China,CN,Beijing
Germany,DE,Berlin
Kenya,KE,Nairobi
When opened in Microsoft Excel looks as below:
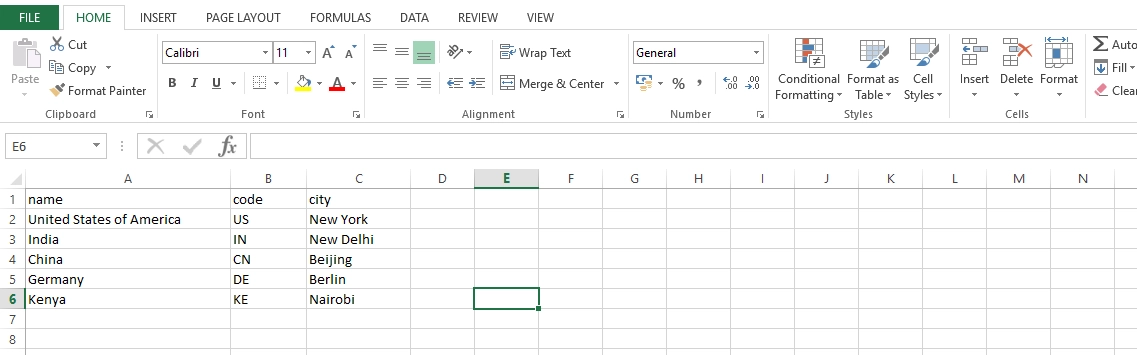
That's it!
By going through this guide, you can effectively generate CSV files from JSON data using PHP.