How to send emails from your website in PHP using SwiftMailer
John Mwaniki / Updated on 07 Jul 2024Swift Mailer is a popular library for sending emails from PHP applications, that is widely accepted by the PHP community.
It is pretty straightforward and simple to use in your projects.
It is a highly flexible and object-oriented open-source library that requires PHP 7.0 or higher to work.
Installation
The recommended way to install Swift Mailer is via Composer
Installation on Windows via Composer
Create a folder where you will place your project files. In this example, we call it “myproject” and place it in the XAMMP's htdocs directory(C:xampp/htdocs/myproject).
Follow these steps:
1). Open the Command prompt
2). Change to the project directory:
cd c:/xampp/htdocs/myproject
3). Execute the install by composer command:
composer require swiftmailer/swiftmailer
When the installation is complete, composer generates an “autoload.php” file within the “vendor” directory which you need to import the Swift Mailer in your project .
In our case this file will be found under “C:xampp/htdocs/myproject/vendor/autoload.php”
You import Swiftmailer as below:
<?php
require_once 'vendor/autoload.php';
Installation on Linux via Composer
The installation on Linux is similar to windows. Follow these steps:
1). Open the terminal
2). Change to the project directory:
cd /opt/lampp/htdocs/myproject
3). Execute the install by composer command:
composer require swiftmailer/swiftmailer
Now you are good to go. Import Swiftmailer into your project by requiring the auto-generated autoloader file:
<?php
require_once 'vendor/autoload.php';
Below is a sample code for sending an email with SwiftMailer.
<?php
require 'vendor/autoload.php';
try {
// Create the SMTP Transport
$transport = (new Swift_SmtpTransport('smtp.hostname', 25, 'tls'))
->setUsername('xxxxxxxx')
->setPassword('xxxxxxxx');
// Create the Mailer using your created Transport
$mailer = new Swift_Mailer($transport);
// Create a message
$message = new Swift_Message();
// Setting email content
$message->setSubject('Demo message using the SwiftMailer library.');
$message->setBody("This is the plain text body of the message.");
$message->addPart('<h1>HTML message version</h1>','text/html');
// Setting from and recipient addresses
$message->setFrom(['sender@gmail.com' => 'sender name']);
$message->addTo('recipient@gmail.com','recipient name');
$message->addCc('recipient@gmail.com', 'recipient name');
$message->addBcc('recipient@gmail.com', 'recipient name');
// Attaching files
$attachment = Swift_Attachment::fromPath('example.xls');
$attachment->setFilename('report.xls');
$message->attach($attachment);
$inline_attachment = Swift_Image::fromPath('nature.jpg');
$message->embed($inline_attachment);
// Sending the message
$mailer->send($message);
echo "Message successfully sent";
} catch (Exception $e) {
echo "Message could not be sent. Error: ".$e->getMessage();
}
Breaking it Down
Importing Swift Mailer
This line imports the Swiftmailer library for use in your PHP script. Make sure to change the path to autoload.php in case your PHP script is not located in the same directory/folder with the vendor folder.
require 'vendor/autoload.php';
Initialize Swift Mailer
Initializing the Swiftmailer is the first thing you need to do after importing it.
You do it by creating the transport object.
The Swift Mailer library supports different transports like SMTP and Sendmail while sending an email.
See below how you can set Swiftmailer to use Sendmail transport in case you don't intend to use SMTP.
$transport = new Swift_SendmailTransport('/usr/sbin/sendmail -bs');
To send emails using SMTP, you need to get and use your credentials for the sender email.
Below are some simple steps to get your SMTP details. I will show how to do it in cPanel though its just the same with the other hosting control panels.
1). Login in to cPanel.
2). Navigate to the Email section and click on the Email Accounts
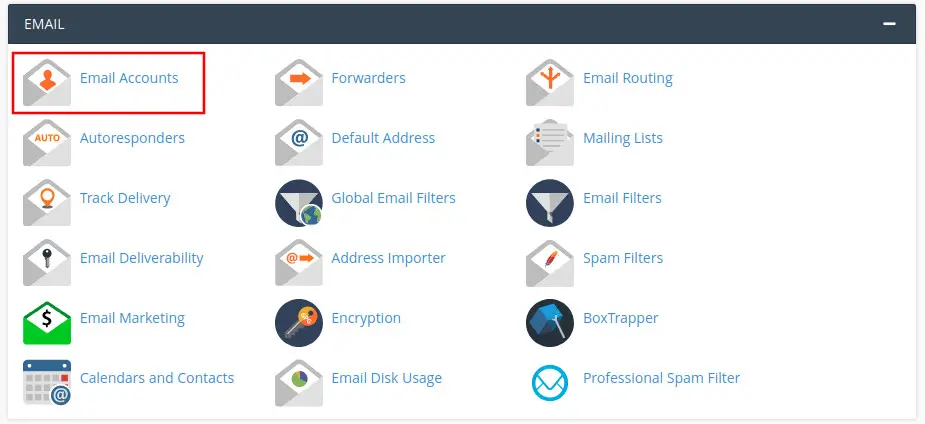
You will see a list of all emails registered with your domain.
If you don't have an email address yet, click on the “ CREATE” button and create one.
3). If you have created emails already, click on the “ CONNECT DEVICES” and you will be directed to a page with the table as below:

What we are most interested with here is the outgoing server hostname(eg. mail.example.com) and SMTP port(eg. 465) because you already have your email/username(email1@example.com) and its password.
The Swift_SmtpTransport() takes three arguments where the first parameter is the SMTP server hostname, the second SMTP port, and the third encryption (either "tls" or "ssl"). The third parameter is optional though some SMTP servers require it in order to work.
$transport = (new Swift_SmtpTransport('mail.example.com', 465, 'ssl'))
->setUsername('email1@example.com')
->setPassword('xxxxxxxx');
Creating and initializing Swiftmailer object
Once the transport is created, we need to initialize a mailer object and pass the transport that we've created already.
$mailer = new Swift_Mailer($transport);
Creating a message
After creating the transport and mailer objects, the only remaining thing is to instantiate the Swift_Message() object and add the various necessary attributes to it.
$message = new Swift_Message();
Setting "from" and "to" addresses
$message->setFrom(['email1@example.com', 'Your Name']);
$message->addTo('johndoe@gmail.com', 'John Doe');
$message->addCc('trump@gmail.com');
$message->addBcc('obama@yahoo.com');
setFrom()
This method takes the sender address as the first parameter, while the second parameter is optional and sets the sender name as will be seen by the recipients.
addTo()
This method is used to set the recipient of the email. Just like setFrom(), it takes the recipient address as the first parameter and the second parameter is the recipient name(optional).
If you want to set multiple recipients, Swiftmailer allows you to use the setTo() method. It takes array arguments.
Setting multiple recipients without names using setTo():
$message->setTo(['johndoe@gmail.com', 'email2@example.com']);
Setting multiple recipients with names using setTo() and associative arrays:
$message->setTo(['johndoe@gmail.com' => 'John Doe', 'email2@example.com' => 'Recipient2 Name']);
The addCc() - CC (carbon-copy) and addBCc() - BCC (blind carbon-copy) are used to set more than one recipient to your email. The differences between the two are that BCC addresses will be invisible to other email recipients.
If you want to have multiple recipients to Cc and BCc, use setCc() and setBcc() respectively. They both take array arguments same as setTo().
$message->setCc(['johndoe@gmail.com' => 'John Doe', 'email2@example.com' => 'Recipient2 Name']);
$message->setBcc(['email1@example.com' => 'Recipient1 Name', 'email2@example.com' => 'Recipient2 Name']);
Attaching files
Swift Mailer allows you to attach files when sending emails. To attach a file, you first need to instantiate the Swift_Attachment object with a valid filename. After creating the attachment object, you can add it to the email with the attach() method. In case you want the recipient to see the file with a different name, you can use the setFilename() method to set the preferred filename.
$attachment = Swift_Attachment::fromPath('example.xls');
$attachment->setFilename('report.xls');
$message->attach($attachment);
At times you may want to attach/embed images within the message body. You do that with the help of the embed() method, as shown in the following snippet. The embed method returns the unique ID of the embedded object, which you can use later on in the message while referencing the image via the src property.
$inline_attachment = Swift_Image::fromPath('imagenme.jpg');
$cid = $message->embed($inline_attachment);
Setting email content
$message->setSubject('Demo message using the SwiftMailer library.');
$message->setBody("This is the plain text body of the message.");
$message->addPart('<h1>HTML message version</h1><p></p>');
The setSubject() method allows you to set the subject of the email.
The setBody() method allows you to set the plain-text version of the message.
The addPart() method allows you to set the HTML version of the message. It takes two parameters where the first parameter is the actual HTML message and the second being content type 'text/html' (optional).
Sending the email
When everything has been set accordingly, it is time to send the email with the following line.
$mailer->send($message);
Examples
Now exploring through the various concepts covered above, below are multiple fully functional examples that you can copy and use in your projects to do tests.
You need to have installed the Swift Mailer within the same directory as your PHP script that you will copy this code into. If you decide to place the file in a different directory please make sure you change “require 'vendor/autoload.php'” to the correct path.
All you need to do is to replace the dummy data with real details
Sending email without SMTP
<?php
require 'vendor/autoload.php';
$transport = new Swift_SendmailTransport('/usr/sbin/sendmail -bs');
$mailer = new Swift_Mailer($transport);
$message = new Swift_Message();
$message->setSubject('Demo message using the SwiftMailer library.');
$message->addPart('<h1>HTML message version</h1>','text/html');
$message->setFrom(['sender@gmail.com' => 'sender name']);
$message->addTo('recipient@gmail.com','recipient name');
if($mailer->send($message)){
echo "<span style='color:green'>Message successfully sent</span>";
}else {
echo "<span style='color:red'>Message could not be sent</span>";
}
?>
Sending email with SMTP
<?php
require_once 'vendor/autoload.php';
$transport = (new Swift_SmtpTransport('mail.example.com', 465, 'ssl'))
->setUsername('sender@gmail.com')
->setPassword('xxxxxxxx');
$mailer = new Swift_Mailer($transport);
$message = new Swift_Message();
$message->setSubject('Demo message using the SwiftMailer library.');
$message->addPart('<h1>HTML message version</h1>','text/html');
$message->setFrom(['sender@gmail.com' => 'sender name']);
$message->addTo('recipient@gmail.com','recipient name');
if($mailer->send($message)){
echo "<span style='color:green'>Message successfully sent</span>";
}else {
echo "<span style='color:red'>Message could not be sent</span>";
}
?>
Sending email from contact form
<?php
$msg = "";
require 'vendor/autoload.php';
if(isset($_POST["submit"])){
$name = $_POST["name"];
$email = $_POST["email"];
$phone = $_POST["phone"];
$subject = $_POST["subject"];
$message = $_POST["message"];
$body = <<<EOD
<!DOCTYPE html>
<html lang="en-US">
<head>
<title>$subject</title>
</head>
<body>
<div style="background-color: #f9f9f9; padding-top: 30px; padding-bottom: 25px;">
<div style="max-width: 640px; margin: 0 auto; box-shadow: 0px 1px 5px rgba(0,0,0,0.1); overflow: hidden; border-top: 4px solid #139401; background: #fff;padding-bottom:25px;">
<table style="width: 640px;" role="presentation" border="0" width="640" cellspacing="0" cellpadding="0" align="center">
<tbody>
<tr>
<td style="padding: 20px;"><span style="font-size: 14pt;"><strong><span style="color: #333232;">Hi Admin!</span></strong></span>
<p><span style="font-family: ’proxima_nova_rgregular’, Helvetica; font-weight: normal; font-size: 12pt; color: #424242;">The message below was sent through contact form:<br /></span></p>
<p><span style="font-family: ’proxima_nova_rgregular’, Helvetica; font-weight: normal; font-size: 12pt; color: #424242;"><span style="color: #52b505;"><strong>Sender Details:</strong></span><br /><span style="color: #262626;">Name</span>: <strong>$name</strong><br /><span style="color: #333232;">Email</span>: <strong>$email</strong> <br />Phone: <strong>$phone</strong></span></p>
<p><span style="font-family: ’proxima_nova_rgregular’, Helvetica; font-weight: normal; font-size: 12pt; color: #424242;"><span style="color: #52b505;"><strong>Subject:</strong></span> <strong>$subject</strong></span></p>
<p><span style="font-family: ’proxima_nova_rgregular’, Helvetica; font-weight: normal; font-size: 12pt; color: #424242;"><span style="color: #52b505;"><strong>Message:</strong></span><br />$message</span></p>
</td>
</tr>
</tbody>
</table>
</div>
</div>
</body>
</html>
EOD;
try{
$transport = (new Swift_SmtpTransport('mail.example.com', 465, 'ssl'))
->setUsername('sender@gmail.com')
->setPassword('xxxxxxxx');
$mailer = new Swift_Mailer($transport);
$message = new Swift_Message();
$message->setSubject($subject);
$message->addPart($body,'text/html');
$message->setFrom(['sender@gmail.com' => 'sender name']);
$message->addTo($email,$name);
$mailer->send($message);
$msg = "<span style='color:green'> Message sent successfully</span>";
} catch (Exception $e) {
$msg = "<span style='color:red'> Message could not be sent.</span>";
}
}
?>
<!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="UTF-8">
<title>Contact Us</title>
<meta name="viewport" content="width=device-width,initial-scale=1">
<style type="text/css">
*{
padding: 0;
margin: 0;
}
.contact{
width: 40%;
display: block;
margin: auto;
margin-top: 20px;
padding: 10px;
box-shadow: 1px 1px 2px;
border-radius: 7px;
background: #f9fcfc;
}
.contact h1{
color: #06062a;
font-size: 28px;
margin: 0px;
padding: 0px;
border-bottom: 1px dashed #ddd;
}
.contact p{
padding: 5px;
}
form{
padding-bottom: 35px;
}
input{
width: 47%;
position: relative;
float: left;
margin-bottom: 10px;
height: 25px;
}
input:nth-child(1),input:nth-child(3){
margin-right: 5px;
}
textarea{
width: 98%;
margin-bottom: 10px;
}
input,textarea{
background: #fff;
border:1px solid #eee;
border-radius: 5px;
padding: 5px;
}
input[type="submit"]{
background: #5ca830;
height: 35px;
color: #fff;
font-size: 16px;
font-weight: 650;
border: none;
border-radius: 8px;
cursor: pointer;
}
#msg{
width: 100%;
}
@media screen and (max-width: 960px){
.contact{
width: 60%;
}
}
@media screen and (max-width: 767px){
.contact{
width: 90%;
}
}
@media screen and (max-width: 640px){
input{
width: 98%;
}
textarea{
width: 98%;
}
}
</style>
</head>
<body>
<div class="contact">
<h1>Contact Form</h1>
<p>All the fields are required</p>
<form action="<?php echo $_SERVER['PHP_SELF']?>" method="post">
<input type="text" name="name" placeholder="Name" required>
<input type="tel" name="phone" placeholder="Phone Number" required>
<input type="email" name="email" placeholder="Email Address" required>
<input type="text" name="subject" placeholder="Message Subject" required>
<textarea name="message" rows="4" placeholder="Write your message here..." required></textarea>
<input type="submit" name="submit" value="Send Message">
<p id="msg"><?php echo $msg;?></p>
</form>
</div>
</body>
</html>
Sending email with details and file attachments from HTML form
This code first uploads the two files into a folder within your project upon clicking the “Submit Details” button, then attaches it to the email.
Create a folder in your project(within the same directory as your PHP script) and name it attachments”.
<?php
$msg = "";
require 'vendor/autoload.php';
if(isset($_POST["submit"])){
$name = $_POST["name"];
$email = $_POST["email"];
$phone = $_POST["phone"];
$subject = $_POST["subject"];
$message = $_POST["message"];
if(is_uploaded_file($_FILES["file1"]["tmp_name"])){
$file1 = $_FILES["file1"]["name"];
$file_tmp1 =$_FILES["file1"]["tmp_name"];
move_uploaded_file($file_tmp1,"attachments/".$file1);
}
if(is_uploaded_file($_FILES["file2"]["tmp_name"])){
$file2 = $_FILES["file2"]["name"];
$file_tmp2 =$_FILES["file2"]["tmp_name"];
move_uploaded_file($file_tmp2,"attachments/".$file2);
}
$body = <<<EOD
<!DOCTYPE html>
<html lang="en-US">
<head>
<title>Contact form submission</title>
</head>
<body>
<p>Hi Admin,<br>
Please find attached some files I have sent you.<br>
My email address is <b>$email</b><br>
Regards,<br>
$name.
</p>
</body>
</html>
EOD;
try{
$transport = (new Swift_SmtpTransport('mail.example.com', 465, 'ssl'))
->setUsername('sender@gmail.com')
->setPassword('xxxxxxxx');
$mailer = new Swift_Mailer($transport);
$message = new Swift_Message();
$message->setFrom(['sender@gmail.com' => 'sender name']);
$message->addTo($email,$name);
$message->setSubject("You have received file attachments");
$message->addPart($body,'text/html');
$message->attach(Swift_Attachment::fromPath("attachments/".$file1));
$message->attach(Swift_Attachment::fromPath("attachments/".$file2));
$mailer->send($message);
$msg = "<span style='color:green'> Message sent successfully</span>";
} catch (Exception $e) {
$msg = "<span style='color:red'> Message could not be sent.</span>";
}
}
?>
<!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="UTF-8">
<title>Attachments Form</title>
<meta name="viewport" content="width=device-width,initial-scale=1">
<style type="text/css">
*{
padding: 0;
margin: 0;
}
.contact{
width: 40%;
display: block;
margin: auto;
margin-top: 20px;
padding: 10px;
box-shadow: 1px 1px 2px;
border-radius: 7px;
background: #f9fcfc;
min-height: 200px;
}
.contact h1{
color: #06062a;
font-size: 28px;
margin: 0px;
padding: 0px;
border-bottom: 1px dashed #ddd;
}
.contact p{
padding: 5px;
}
input{
width: 47%;
position: relative;
float: left;
margin-bottom: 10px;
height: 25px;
}
input:nth-child(1),input:nth-child(3){
margin-right: 5px;
}
input{
background: #fff;
border:1px solid #eee;
border-radius: 5px;
padding: 5px;
}
input[type="submit"]{
background: #5ca830;
height: 35px;
color: #fff;
font-size: 16px;
font-weight: 650;
border: none;
border-radius: 8px;
cursor: pointer;
}
#msg{
width: 100%;
}
@media screen and (max-width: 960px){
.contact{
width: 60%;
}
}
@media screen and (max-width: 767px){
.contact{
width: 90%;
}
}
@media screen and (max-width: 640px){
input{
width: 98%;
}
textarea{
width: 98%;
}
}
</style>
</head>
<body>
<div class="contact">
<h1>Attachments Form</h1>
<p>All the fields are required</p>
<form action="<?php echo $_SERVER['PHP_SELF']?>" method="post" enctype="multipart/form-data">
<input type="text" name="name" placeholder="Name" required>
<input type="email" name="email" placeholder="Email Address" required>
<input type="file" name="file1" accept=".jpg,.png,.pdf,.docx,.jpeg" required>
<input type="file" name="file2" accept=".jpg,.png,.pdf,.docx,.jpeg" required>
<input type="submit" name="submit" value="Submit Details">
<p id="msg"><?php echo $msg;?></p>
</form>
</div>
</body>
</html>
Conclusion
In this tutorial, you learned what SwiftMailer is, how to install it in your PHP project, and how you can use it to send emails with or without SMTP. You also learned how to send emails to multiple recipients, with or without attachments as well as how to collect and send HTML form information via email using SwiftMailer.